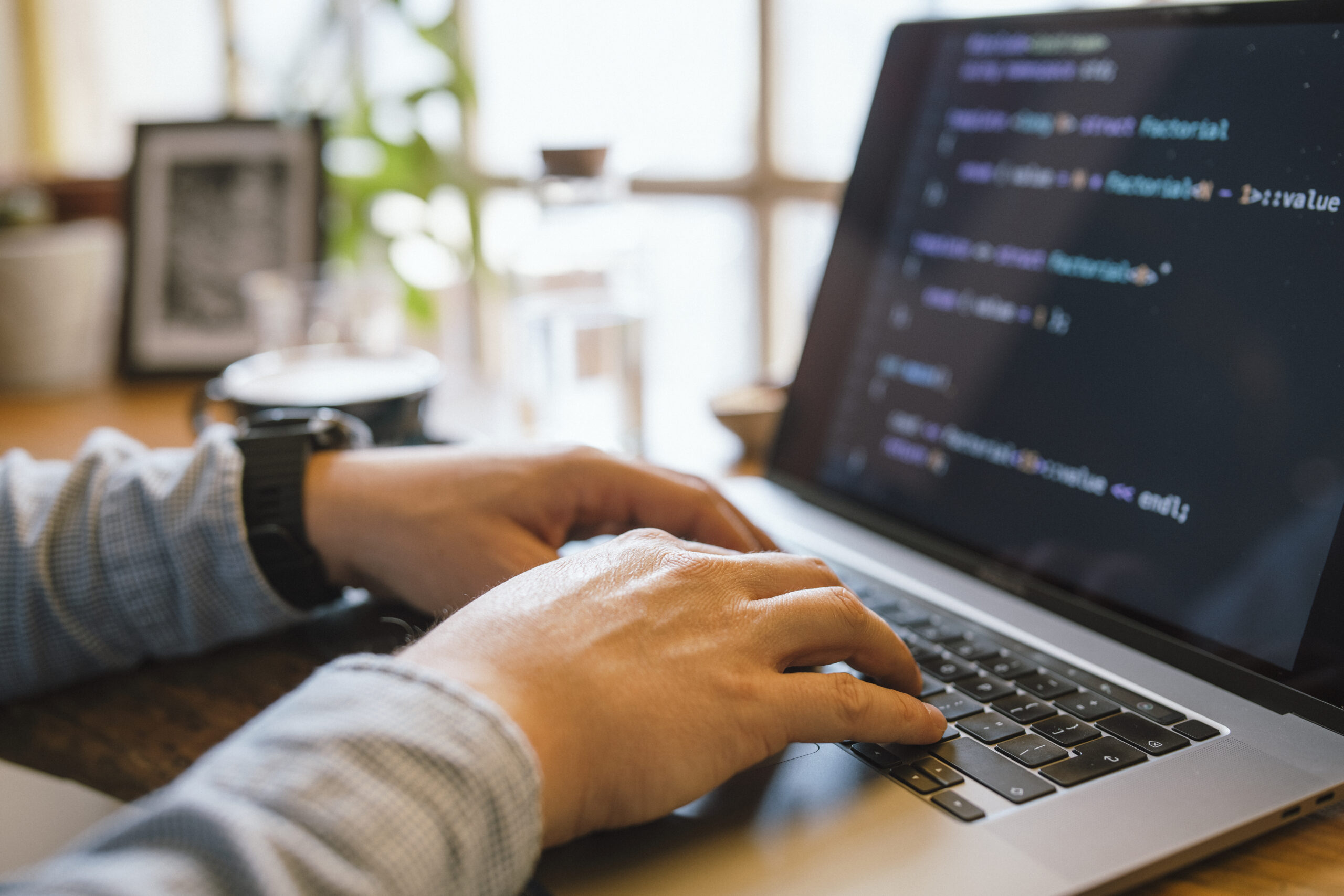
Debugging is Just about the most vital — nonetheless frequently disregarded — capabilities in a very developer’s toolkit. It isn't really just about fixing broken code; it’s about comprehending how and why items go Improper, and Finding out to Consider methodically to resolve challenges successfully. Irrespective of whether you are a rookie or maybe a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably transform your productiveness. Allow me to share many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of growth, understanding how to connect with it properly throughout execution is Similarly critical. Modern day development environments occur Outfitted with powerful debugging abilities — but several builders only scratch the floor of what these tools can perform.
Get, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code about the fly. When utilised correctly, they Permit you to notice just how your code behaves during execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclusion developers. They permit you to inspect the DOM, observe community requests, check out real-time effectiveness metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can convert frustrating UI troubles into workable tasks.
For backend or technique-amount builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control about running procedures and memory administration. Mastering these tools could have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Outside of your IDE or debugger, turn out to be relaxed with Variation Command methods like Git to grasp code record, obtain the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your instruments signifies likely outside of default configurations and shortcuts — it’s about acquiring an personal knowledge of your development atmosphere in order that when concerns come up, you’re not dropped at nighttime. The higher you recognize your instruments, the more time it is possible to commit fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and infrequently forgotten — techniques in powerful debugging is reproducing the challenge. Ahead of jumping into the code or earning guesses, builders need to have to create a consistent ecosystem or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Talk to inquiries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered more than enough data, try to recreate the situation in your local setting. This could indicate inputting the same knowledge, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automated exams that replicate the sting cases or condition transitions included. These tests not merely assistance expose the issue and also prevent regressions Later on.
From time to time, the issue could be natural environment-specific — it might come about only on sure operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for endurance, observation, and also a methodical solution. But when you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible circumstance, You may use your debugging tools more successfully, check possible fixes securely, and communicate much more clearly together with your group or customers. It turns an abstract complaint right into a concrete obstacle — Which’s the place developers prosper.
Read through and Recognize the Error Messages
Error messages tend to be the most valuable clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, developers must discover to take care of mistake messages as direct communications in the system. They normally inform you what exactly occurred, where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking through the message carefully As well as in total. Many builders, especially when less than time force, glance at the main line and quickly begin making assumptions. But further within the mistake stack or logs could lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and recognize them initial.
Split the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it stage to a selected file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you towards the accountable code.
It’s also practical to comprehend the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and learning to recognize these can greatly quicken your debugging approach.
Some faults are vague or generic, and in those situations, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase with the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging levels include DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information during enhancement, Details for standard functions (like productive begin-ups), Alert for probable troubles that don’t break the application, Mistake for true issues, and FATAL if the technique can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Focus on vital functions, state variations, input/output values, and critical final decision points inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. By using a well-imagined-out logging tactic, you are able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and Enhance the Total maintainability and trustworthiness of your code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it's a sort of investigation. To correctly determine and correct bugs, builders ought to approach the process like a detective solving a thriller. This frame of mind can help stop working complex problems into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much relevant info as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire yourself: What could be leading to this behavior? Have any changes a short while ago been built to your codebase? Has this situation transpired prior to under identical situation? The purpose is always to narrow down alternatives and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled natural environment. In case you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, talk to your code issues and Allow the effects direct you nearer to the truth.
Pay shut interest to compact information. Bugs often cover within the the very least anticipated sites—just like a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and affected person, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes might cover the real dilemma, just for it to resurface later.
And finally, keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run issues and aid Some others comprehend your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical abilities, tactic complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Produce Checks
Writing tests is one of the best strategies to help your debugging skills and General advancement effectiveness. Assessments not simply assistance capture bugs early but will also function a security Web that gives you confidence when creating adjustments in your codebase. A properly-examined application is much easier to debug mainly because it helps you to pinpoint accurately where and when a problem takes place.
Get started with device assessments, which center on particular person features or modules. These modest, isolated assessments can swiftly reveal regardless of whether a particular piece of logic is working as envisioned. Any time a take a look at fails, you quickly know the place to search, considerably decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Formerly becoming fixed.
Future, combine integration tests and end-to-conclusion assessments into your workflow. These assist ensure that many areas of your application do the job collectively smoothly. They’re significantly valuable for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your assessments can let you know which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To check a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge scenarios. This degree of knowledge By natural means potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails regularly, you may focus on repairing the bug and enjoy your exam pass when The problem is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In brief, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the trouble—watching your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your head, decrease disappointment, and sometimes see The problem from a new viewpoint.
When you're also near to the code for also extended, cognitive tiredness sets in. You could possibly start out overlooking evident glitches or misreading code you wrote just several hours previously. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, especially all through extended debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to maneuver all around, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weak point—it’s a wise system. It provides your Mind House to breathe, improves your viewpoint, and allows you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Every single Bug
Every bug you experience is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you make the effort to replicate and analyze what went Incorrect.
Commence by asking by yourself a number of critical thoughts as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers often reveal blind places in the workflow or being familiar with and help you build much better coding patterns going ahead.
Documenting bugs can even be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, assisting others stay away from the exact same issue boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In more info the end, many of the very best builders aren't those who create best code, but those that repeatedly learn from their problems.
In the end, Every single bug you fix adds a different layer for your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.